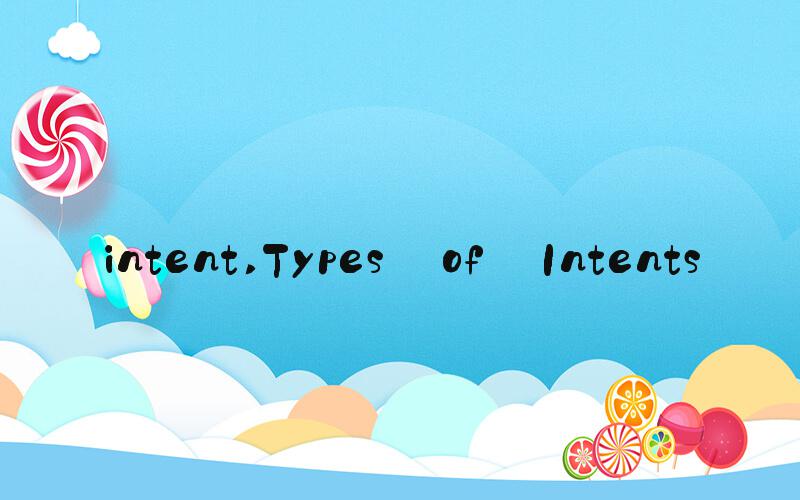
Intent is a concept in Android programming that allows developers to create and execute activities, services, and broadcasts in their applications. It is used to communicate between different components of the same or different applications. When an intent is created, it is used to define the what, why, and how of a particular action. In this article, we will explore the different types of intents, their uses, and how they can be implemented in Android programming.
Types of IntentsThere are two types of intents in Android programming: Explicit Intents and Implicit Intents.
Explicit IntentsExplicit Intents are used to start an activity, perform a service, or broadcast to an activity within the same application. An Explicit Intent specifies the component's name, ensuring that the intent is sent to the correct component when executed. Explicit Intents are commonly used to navigate between screens in the application.
Implicit IntentsImplicit Intents are used to invoke system services or activities outside the application. They do not specify the component's name but instead describe the action to be performed by the receiving application. Implicit Intent is used when you want to start an activity, for example, from another application or open an email client. So, if you don't know which application to use to perform an action, then you can choose Implicit Intents.
Uses of IntentsIntents are used in Android programming for a variety of purposes, including:
Starting a new activity or service
Sending data between activities or services
Starting an activity of another application
Broadcasting a message within an application or to other applications
Starting a system service like the camera or phone app
Implementing Intents in AndroidTo implement an Intent in an Android application, there are a few key steps:
Create an Intent object:
In the first step, you create an Intent object by specifying the context of the current activity and the class you want to start, or the action you want to perform. Here is an example of how to create an Explicit Intent:
Intent intent = new Intent(this, OtherActivity.class);
startActivity(intent);
Here is an example of how to create Anonymous Implicit Intent:
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse("http://www.google.com"));
startActivity(intent);
Start an activity or service:
In the next step, you start the Intent using the startActivity() method. Here is an example of how to start an Explicit Intent:
startActivity(intent);
Here is an example of how to start Anonymous Implicit Intent:
startActivity(Intent.createChooser(intent, "Open with"));
Sending data between activities:
You can use Intents to send data between activities by adding extra information to the intent using the putExtra() method. Here is an example:
Intent intent = new Intent(this, SecondActivity.class);
intent.putExtra("key", value);
startActivity(intent);
To retrieve the passed data in the SecondActivity, you can use the getIntent() method and get the extras with the getExtras() method. Here is an example:
Intent intent = getIntent();
String value = intent.getExtras().getString("key");
Broadcasting messages:
To send a message to a BroadcastReceiver, you can create an intent and call the sendBroadcast() or sendOrderedBroadcast() method. Here is an example:
Intent intent = new Intent("custom.intent.action.MESSAGE");
intent.putExtra("message", "Hello World");
sendBroadcast(intent);
To receive the broadcast message, you create a BroadcastReceiver and register it with an IntentFilter that matches the intent filter used for sending the broadcast in the sendBroadcast() or sendOrderedBroadcast() method. Here is an example:
public class MyReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
String message = intent.getStringExtra("message");
// Do something with the message
}
}
Conclusion
Intents are a powerful tool in Android programming that allow developers to create and execute activities, services, and broadcasts in their applications. There are two types of intents, Explicit Intents and Implicit Intents, which can be used to start an activity, perform a service, or broadcast to an activity within the same application or outside the application. Intents are used to communicate between different components of the same or different applications and can be implemented using a few key steps. By understanding how to use Intents, developers can create more robust and dynamic Android applications.